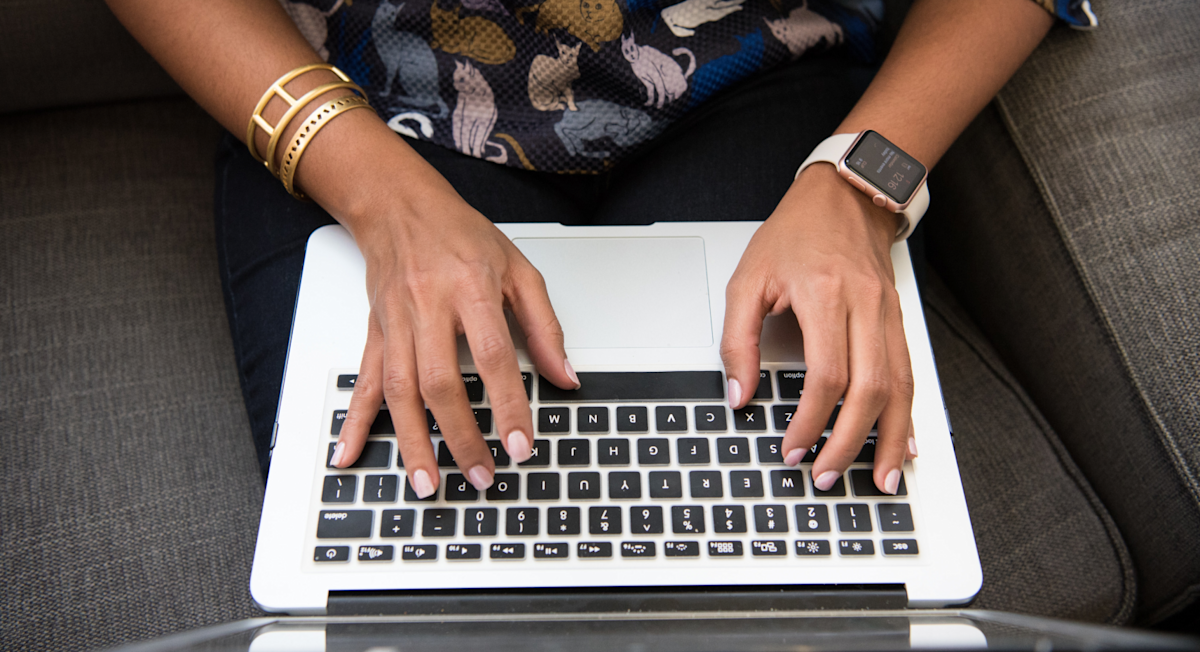
Create React App is a powerful tool for building React applications with zero configuration. You should not install the environment and tune Webpack, Babel, and other necessary tools; you can write code instantly without any distress.
Create React App is a powerful tool for building React applications with zero configuration. You should not install the environment and tune Webpack, Babel, and other necessary tools; you can write code instantly without any distress.
Free code review checklist and best practices
DownloadCreate React App is a powerful tool for building React applications with zero configuration. You should not install the environment and tune Webpack, Babel, and other necessary tools; you can write code instantly without any distress.
Let's go ahead and install the boilerplate.
npm install -g create-react-app
create-react-app my-app
cd my-app/
npm start
That is all! Now you can write business logic for your application.
Create React App is a tool created by React.js developers. These guys really know how the app should work.
You can quickly update your configuration. Just change the react-scripts version in your package.json file.
There are no unnecessary files like .rc files or webpack.config or something else. Your app folder is much cleaner than usual.
It is stable. Extremely stable. It will work on each OS and each environment. You will not waste your time trying to fix your crashing app.
It is extendable. There are a lot of plugins and extensions so that you can build your own environment.
If you want to customize your webpack configuration and run npm run eject, you will not receive configuration updates. Also, it will move all config files to your project folder.
It does not support server-side rendering out-of-the-box.
You can find a package.json file in the project's root folder. It contains the project info and a list of dependencies that are located in the "/node_modules" folder and can be installed by the "npm install" command, and a list of scripts that can be run with "npm run" command. There are four scripts by default: "start" runs the application in development mode; "build" assembles the production files; "test" runs the unit tests. Finally, the "eject" script unpacks the react-scripts to the project folder, do not use it if you want to receive updates.
The next important part is the "/public" folder. A user can access it. Here you can find an index.html file to change app layout, page title, and favicon.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no">
<meta name="theme-color" content="#000000">
<!--
manifest.json provides metadata used when your web app is added to the
homescreen on Android. See https://developers.google.com/web/fundamentals/engage-and-retain/web-app-manifest/
-->
<link rel="manifest" href="%PUBLIC_URL%/manifest.json">
<link rel="shortcut icon" href="%PUBLIC_URL%/favicon.ico">
<!--
Notice the use of %PUBLIC_URL% in the tags above.
It will be replaced with the URL of the `public` folder during the build.
Only files inside the `public` folder can be referenced from the HTML.
Unlike "/favicon.ico" or "favicon.ico", "%PUBLIC_URL%/favicon.ico" will
work correctly both with client-side routing and a non-root public URL.
Learn how to configure a non-root public URL by running `npm run build`.
-->
<title>React App</title>
</head>
<body>
<noscript>
You need to enable JavaScript to run this app.
</noscript>
<div id="root"></div>
<!--
This HTML file is a template.
If you open it directly in the browser, you will see an empty page.
You can add webfonts, meta tags, or analytics to this file.
The build step will place the bundled scripts into the <body> tag.
To begin the development, run `npm start` or `yarn start`.
To create a production bundle, use `npm run build` or `yarn build`.
-->
</body>
</html>
Your app's source is placed in the "/src" folder; you will write your code here. Also, here you can see how to interact with styles, images, and other assets. The entry point is index.js, where we import and mount our app to the div with "root" id via ReactDom library. Also, here we import styles from index.css. Usually, they are global styles; I recommend importing small CSS files for each component. It will help make the components reusable.
import React from 'react';
import ReactDOM from 'react-dom';
import './index.css';
import App from './App';
import registerServiceWorker from './registerServiceWorker';
ReactDOM.render(, document.getElementById('root'));
registerServiceWorker();
The next is App.js. It is a React component; here, you can view JSX markup. The other important thing to know is how to import and render images. Here, you can see an example in logo.svg.
import React, { Component } from 'react';
import logo from './logo.svg';
import './App.css';
class App extends Component {
render() {
return (
<div className="App">
<header className="App-header">
<img src={logo} className="App-logo" alt="logo" />
<h1 className="App-title">Welcome to React</h1>
</header>
<p className="App-intro">
To get started, edit <code>src/App.js</code> and save to reload.
</p>
</div>
);
}
}
export default App;
Finally, we have App.test.js.
import React from 'react';
import ReactDOM from 'react-dom';
import App from './App';
it('renders without crashing', () => {
const div = document.createElement('div');
ReactDOM.render(<App />, div);
});
Here, you can write unit tests and ensure that your app works correctly. I also recommend creating one file for each component if you need tests, of course.
These are the basics of Create React App. In the next article, we will talk about React Router.
You also might be interested in checking out this case study.
VT Labs does agile development for Shopify stores, to move faster than competitors. You get store improvements every week and can change priorities anytime.