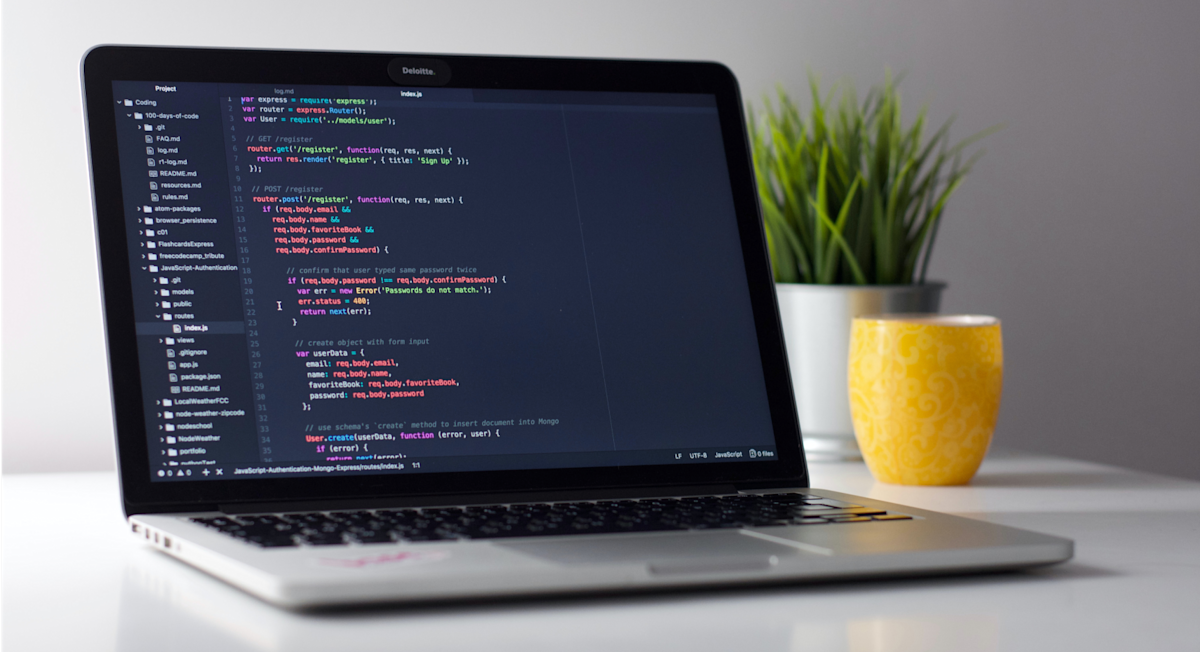
We have already created a simple app via Create React App boilerplate. Now, let's go on with the React Router tutorial.
We have already created a simple app via Create React App boilerplate. Now, let's go on with the React Router tutorial.
Free code review checklist and best practices
DownloadReact Router is a library that allows for the creation of navigation for the React app. You can declare multiple URLs and handle their changes. React Router provides two versions of the library to maintain navigation for React web apps and React Native mobile apps. We should use the "react-router-dom" package for our app. Let's add it to our package.json file and run an npm install.
npm i react-router-dom --save
The current version of the router is 4.x.x; there is a big difference between 3.x.x and the recent releases so that we will use the new one.
Let's create the "Components" folder with four files: IndexComponent.js, PageComponent.js, NotFoundComponent.js, and NavigationComponent.js.
The "Views" and navigation menus will be inside our route URLs. Here is "Views":
IndexComponent.js
import React, { PureComponent } from 'react';
class IndexComponent extends PureComponent {
render() {
return (
<div>
<h1>This is a home page</h1>
</div>
);
}
}
export default IndexComponent;
PageComponent.js
import React, { PureComponent } from 'react';
class PageComponent extends PureComponent {
render() {
return (
<div>
<h1>This is the page number {this.props.match.params.number}</h1>
</div>
);
}
}
export default PageComponent;
NotFoundComponent.js
import React, { PureComponent } from 'react';
class NotFoundComponent extends PureComponent {
render() {
return (
<div>
<h1>Page Not Found!</h1>
</div>
);
}
}
export default NotFoundComponent
;Also, here is our NavigationComponent. Notice that we use NavLink to implement router links. This component from React Router allows for navigation across the routes without page reloading. Also, NavLink has an "active" class in it if the route matches, so we can add some styles to active links if we want.
NavigationComponent.js
import React, { Component } from 'react';
import { NavLink } from 'react-router-dom';
class NavigationComponent extends Component {
render() {
return (
<div>
<NavLink exact to="/">Home</NavLink>
<NavLink to="/page/2">Second</NavLink>
</div>
);
}
}
export default NavigationComponent;
Now we should put it all together and create our routes in the App.js file. We will use the BrowserRouter component to define the Router, Switch component that allows selecting what path we should render, and the Route component, which will receive the element.
App.js
import React, { Component } from 'react';
import { BrowserRouter, Switch, Route } from 'react-router-dom';
import IndexComponent from './Components/IndexComponent';
import PageComponent from './Components/PageComponent';
import NavigationComponent from './Components/NavigationComponent';
import NotFoundComponent from './Components/NotFoundComponent';
import logo from './logo.svg';
import './App.css';
class App extends Component {
render() {
return (
<div className="App">
<header className="App-header">
<img src={logo} className="App-logo" alt="logo" />
<h1 className="App-title">Welcome to React</h1>
</header>
<BrowserRouter>
<div>
<NavigationComponent />
<Switch>
<Route exact path="/" component={IndexComponent} />
<Route exact path="/page/:number" component={PageComponent} />
<Route component={NotFoundComponent} />
</Switch>
</div>
</BrowserRouter>
</div>
);
}
}
export default App;
Notice that we have "exact" prop in the index route that tells the router not to render the home page component if the URL has subroutes. Also, we have the 'number' param in the second route that appears as 'this.props.match.params.number' inside the PageComponent.js.
We have created a navigation system for our app, including a Homepage, Second page, and 404 page if we navigate the wrong URL. Screenshots of how it should look following:
If you're curious, this article explores why the software development life cycle (SDLC) methodology can be harmful.
VT Labs does agile development for Shopify stores, to move faster than competitors. You get store improvements every week and can change priorities anytime.